As we step into the future of technology, we find ourselves in an era where blockchain is gradually gaining widespread acceptance and transforming the digital world. Now, imagine combining this revolutionary technology with an efficient and robust programming language.
The outcome is nothing short of remarkable – a Rust blockchain. Rust, renowned for its speed, reliability, and performance, presents itself as a potent tool for crafting intricate blockchains.
We’ll take you through a fascinating journey, a step-by-step process to create your own blockchain using Rust. It’s about time we bridge the gap between possibility and reality.
Contents
- 1 Unraveling Rust: An Ideal Tool for Blockchain
- 2 Using Rust to Create Blockchain
- 2.1 1. Setting up Rust
- 2.2 2. Structuring your Block
- 2.3 3. Defining your block properties
- 2.4 4. Implementing the Block Constructor
- 2.5 5. Debugging
- 2.6 6. Returning to main.rs
- 2.7 7. Creating a Hashable Trait
- 2.8 8. Use Hashable on the Block
- 2.9 9. Make a Blockchain Structure
- 2.10 10. Mining
- 2.11 11. Blockchain Validation
- 3 Why should Blockchain Developers use Rust?
- 4 Some Potential Challenges with Rust
- 5 Final Words
Unraveling Rust: An Ideal Tool for Blockchain
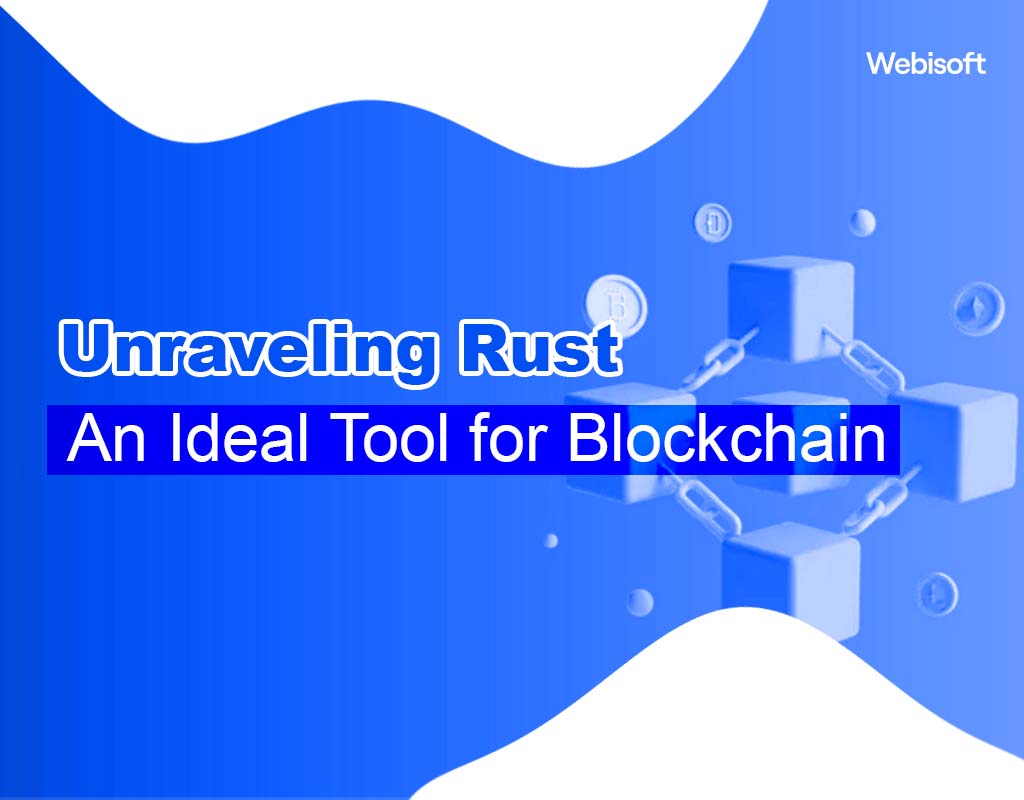
Imagine a coding language that’s specifically crafted for blockchain development, a space where trustworthiness and performance take center stage. Enter Rust, a programmer’s dream recognized for its speed, dependability, and memory economy.
Given the escalating interest in blockchain technology, this write-up aims to provide a methodical, easy-to-follow blockchain guide on constructing a blockchain using Rust.
For simplicity, we’ll stick to a very basic and generic implementation of Rust blockchain.
Why Choose Rust?
Rust, a dynamically compiled language, boasts a rich type system and a unique concept of ownership. What makes it stand out is its speed and memory efficiency. It can detect and fix errors during compilation, a feature incredibly useful to developers building high-performing, critical applications.
All while ensuring memory and thread safety.
Rust comes fully equipped with comprehensive documentation, a user-friendly compiler, and top-tier tools. These include integrated package managers and versatile editors that can handle tasks like type inspection and auto-completion with ease.
The Charm of Rust
What makes Rust so compelling is its robustness. It’s designed to prevent crashes, offering a level of security that puts it in the same league as JavaScript, Ruby, and Python.
But Rust takes it a step further. It allows you to write flawless parallel code, something that’s just not feasible with C/C++. With Rust, you get a versatile tool that can accurately emulate different programming paradigms swiftly and efficiently.
Using Rust to Create Blockchain
Building a blockchain with Rust can be an exciting journey, bringing together the powers of decentralization and the robustness of a systems programming language. Let’s walk through it step by step.
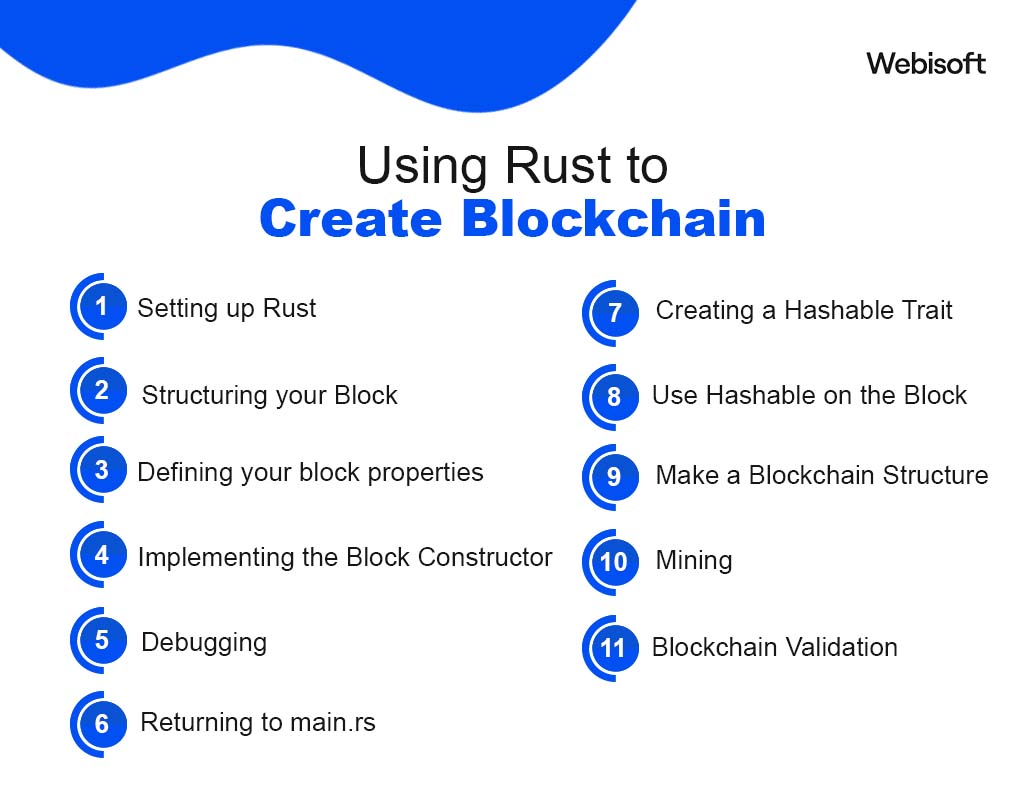
1. Setting up Rust
Before you dive in, ensure you’ve installed Rust on your system. If you haven’t, you can get it from the official Rust website. Remember, Rust comes with “rustup” which is a toolchain installer and manager.
After installation, confirm its presence by typing “rustc –version” on your command line.
2. Structuring your Block
In Rust, we create ‘structs’ that define what a particular entity looks like. To start, create two source files: main.rs and block.rs.
The main.rs file will act as your central hub for running your application while the block.rs file will help you define your block.
3. Defining your block properties
In your block.rs file, you need to define the properties of a block. These properties could include elements like the block hash, timestamp, previous block hash, and transaction data.
pub struct Block { pub index: u32, pub timestamp: u128, pub hash: Hash, pub prev_block_hash: Hash, pub nonce: u64, pub transactions: Vec, pub difficulty: u128,}
4. Implementing the Block Constructor
After defining your block properties, you’ll need to create a block constructor.
impl Block { pub fn new (index: u32, timestamp: u128, prev_block_hash: Hash, transactions: Vec, difficulty: u128) -> Self { Block { index, timestamp, hash: vec![0; 32], prev_block_hash, nonce: 0, transactions, difficulty, } }
This is a function that will allow you to create new instances of your block with specific properties.
5. Debugging
Adding a debug formatter to the block can assist you in troubleshooting and tracking the progress of your info throughout development.
impl Debug for Block { fn fmt (&self, f: &mut Formatter) -> fmt::Result { write!(f, “Block[{}]: {} at: {} with: {} nonce: {}”, &self.index, &hex::encode(&self.hash), &self.timestamp, &self.transactions.len(), &self.nonce, ) }}
You can use Rust’s inbuilt fmt::Debug trait for this purpose.
6. Returning to main.rs
Once you’ve defined your block and its features, head back to main.rs.
fn main () { let difficulty = 0x000fffffffffffffffffffffffffffff; let mut genesis_block = Block::new(0, now(), vec![0; 32], vec![ Transaction { inputs: vec![ ], outputs: vec![ transaction::Output { to_addr: “Alice”.to_owned(), value: 50, }, transaction::Output { to_addr: “Bob”.to_owned(), value: 7, }, ], }, ], difficulty);
This is where you’ll tie everything together, creating new instances of your blocks and adding them to the blockchain.
7. Creating a Hashable Trait
In a new file named hashable.rs, create a trait named ‘Hashable’. Traits in Rust are a way of grouping method signatures together that describe a particular piece of behavior.
use super::*;pub trait Hashable { fn bytes (&self) -> Vec; fn hash (&self) -> Hash { crypto_hash::digest(crypto_hash::Algorithm::SHA256, &self.bytes()) }}
The hashable.rs file will allow you to create a hash for every block, a critical component of the blockchain’s security.
8. Use Hashable on the Block
Let us presently write a new “impl” application block to set up the bytes method on the previously generated block struct.
impl Hashable for Block { fn bytes (&self) -> Vec { let mut bytes = vec![]; bytes.extend(&u32_bytes(&self.index)); bytes.extend(&u128_bytes(&self.timestamp)); bytes.extend(&self.prev_block_hash); bytes.extend(&u64_bytes(&self.nonce)); bytes.extend( self.transactions .iter() .flat_map(|transaction| transaction.bytes()) .collect::<Vec>() ); bytes.extend(&u128_bytes(&self.difficulty)); bytes }}
9. Make a Blockchain Structure
Make a new file called “blockchain.rs” in which to build your fresh blockchain structure.
pub struct Blockchain {
Since it’s a block vector, we should add its field.
pub blocks: Vec,
10. Mining
Let’s dive into the process of mining, you’ll want to navigate to the file titled “main.rs”. For our example, let’s say we want to mine 10 blocks until we reach our target count. Let’s call this the genesis block. It’s critical to ensure that each block has its unique hash as well as the hash of its predecessor.
fn main () { let difficulty = 0x000fffffffffffffffffffffffffffff; let mut block = Block::new(0, 0, vec![0; 32], 0, “Genesis block!”.to_owned(), difficulty); block.mine(); println!(“Mined genesis block {:?}”, &block); let mut last_hash = block.hash.clone(); let mut blockchain = Blockchain{ block: vec![block], }; for i in 1..=10 { let mut block = Block::new(i, 0, last_hash, 0, “Another block!”.to_owned(), difficulty); block.mine(); println!(“Mined block {:?}”, &block); last_hash = block.hash.clone(); blockchain.blocks.push(block);
Upon executing these codes, you will successfully generate a blockchain structure that incorporates 10 unique blocks.
11. Blockchain Validation
To validate the blockchain, add the subsequent method to the ledger struct inside blockchain.rs.
impl Blockchain { pub fn new () -> Self { Blockchain { blocks: vec![], unspent_outputs: HashSet::new(), } }pub fn update_with_block (&mut self, block: Block) -> Result<(), BlockValidationErr> { let i = self.blocks.len(); if block.index != i as u32 { return Err(BlockValidationErr::MismatchedIndex); } else if !block::check_difficulty(&block.hash(), block.difficulty) { return Err(BlockValidationErr::InvalidHash); } else if i != 0 { // Not genesis block let prev_block = &self.blocks[i – 1]; if block.timestamp <= prev_block.timestamp { return Err(BlockValidationErr::AchronologicalTimestamp); } else if block.prev_block_hash != prev_block.hash { return Err(BlockValidationErr::MismatchedPreviousHash); } } else { // Genesis block if block.prev_block_hash != vec![0; 32] { return Err(BlockValidationErr::InvalidGenesisBlockFormat); } }
Each of these steps is just a snapshot of the process. In actual blockchain development, complex algorithms are set up, transaction systems are built, and network communication is ensured. But by following these steps, you’re on your way to creating a fundamental blockchain with Rust.
Why should Blockchain Developers use Rust?
Choosing the right language for blockchain development is as crucial as understanding the technology itself.
Rust, a modern systems programming language, is quickly becoming the language of choice for many developers working on blockchain projects. Here’s why:
[Note for Designer: Please do an infographic here]
High-Level Performance
Rust is designed to provide the same level of performance as low-level languages like C++ and C, yet it also incorporates high-level abstractions similar to those found in languages like Python.
Rust can execute complex tasks quickly, which is crucial for blockchain technology which needs to handle many transactions per second.
Safety
Rust’s focus on safety makes it an attractive choice for blockchain development. It’s designed to prevent common programming errors like null pointer dereferencing, buffer overflows, and data races.
By eliminating these issues, Rust enhances the reliability and security of the blockchain.
Memory-Efficient
Rust’s strict compile-time checks and lack of a garbage collector ensure efficient memory usage. In a blockchain network, where numerous nodes may be running the blockchain, efficient memory use can be a significant advantage.
Interoperability with C++ and C
Rust can interoperate with C++ and C, allowing developers to leverage existing C++ and C libraries in their Rust blockchain code. This interoperability feature makes Rust an adaptable and flexible choice for blockchain development.
No Runtime Indeterminism
Because Rust lacks a garbage collector, there is no unpredictability or ‘indeterminism’ introduced by the language at runtime. In a blockchain system, where determinism is essential for consensus across the network, this feature is particularly valuable.
Given these attributes, Rust aligns very well with the demands of blockchain development where efficiency, reliability, and performance are paramount.
Are you considering a bespoke blockchain built in Rust for your company? Webisoft’s seasoned team of blockchain professionals is ready to assist. We can guide you through the intriguing topic of blockchain if you’re developing a new solution or incorporating old blockchain technology.
Some Potential Challenges with Rust
While Rust offers a multitude of benefits for software development, it does present some hurdles to developers:
The Stickler Compiler
Rust’s compiler is notorious for being unyielding when it comes to memory-related issues. It’s particularly stringent about cleaning up memory calls, which can sometimes feel a tad too restrictive.
A Different Approach to OOP
If you’re a developer who loves classic OOP-style inheritance and classes, Rust might throw you for a loop. The language doesn’t provide typical classes and inheritance models familiar to many programmers.
Final Words
To recap, we’ve embarked on an enlightening journey, demystifying the process of creating a blockchain using Rust. We delved into the rich capabilities of Rust, highlighting its dynamic nature, memory efficiency, and superior toolsets.
Moreover, we explored how Rust provides an edge over other languages, particularly in writing flawless parallel code. Lastly, we walked you through the hands-on process of mining your own blocks.
The magic of the Rust blockchain isn’t just in its advanced capabilities, but also in its accessibility to developers, whether you’re a seasoned programmer or just starting out in the blockchain universe.Ready to transform your ideas into reality with cutting-edge technology? Connect with Webisoft, a leader in custom software, and blockchain development. Our experts are highly proficient in using Rust, Python, and other languages. Whether it’s dApp, mobile, or web development, our expert team has got you covered.