Did you know that the global NFT market reached $22 billion in trading volume in 2021? The Non-Fungible Tokens (NFTs) are experiencing a remarkable transformation. ERC-721 tokens lead the way as the standard for representing ownership of unique digital assets on the Ethereum blockchain.
But what does the ERC 721 Tokens offer?
ERC-721 tokens provide a secure and transparent way to prove ownership of unique assets. Their flexibility allows creators to add extra features. They work seamlessly on various platforms, ensuring transparent and protected digital ownership, and this sets the stage for innovative applications.
That’s just the beginning. Stick with us, as we delve into the features, benefits, real-world applications, and the process of creating ERC 721 tokens. We’ll also introduce you to Webisoft, a game-changer that specializes in assisting with ERC-721 token development and implementation.
Let’s start!
Contents
- 1 What is ERC721?
- 2 How does the ERC-721 standard work?
- 3 Benefits of Token ERC-721 for NFTs
- 4 What Are The Steps To Create and Deploy Erc 721 Tokens?
- 4.1 Step 1: Planning Your ERC-721 Token
- 4.2 Step 2: Setting Up Development Environment
- 4.3 Step 3: Writing Smart Contract for ERC-721 Token
- 4.4 Step 4: Compiling and Deploying the Smart Contract
- 4.5 Step 5: Testing and Debugging
- 4.6 Step 6: Front-End Integration
- 4.7 Step 7: Security Considerations
- 4.8 Step 8: Deployment and Maintenance
- 5 8 Use Cases for ERC-721 NFTs
- 6 Real-world examples of successful projects utilizing ERC-721 tokens
- 7 How to create NFT solidity With ERC-721?
- 8 How Webisoft Helps with erc721 token development and Implementation
- 9 Conclusion
- 10 FAQs
- 10.1 Are ERC-721 tokens compatible with other blockchain networks besides Ethereum?
- 10.2 How can I ensure the uniqueness of my ERC-721 tokens in a large collection?
- 10.3 Is it possible to change the metadata associated with an existing ERC-721 token?
- 10.4 Can ERC-721 tokens represent physical assets in the real world?
What is ERC721?
ERC721 is a standard for representing non-fungible tokens (NFTs) on the Ethereum blockchain. Unlike fungible tokens, like cryptocurrencies, where each token is identical and interchangeable, non-fungible tokens are unique and can represent ownership of specific assets or items.
The ERC721 standard defines a set of rules and functions that a smart contract must implement to manage these unique tokens, allowing for the creation, transfer, and querying of ownership details.
Functions of ERC-721
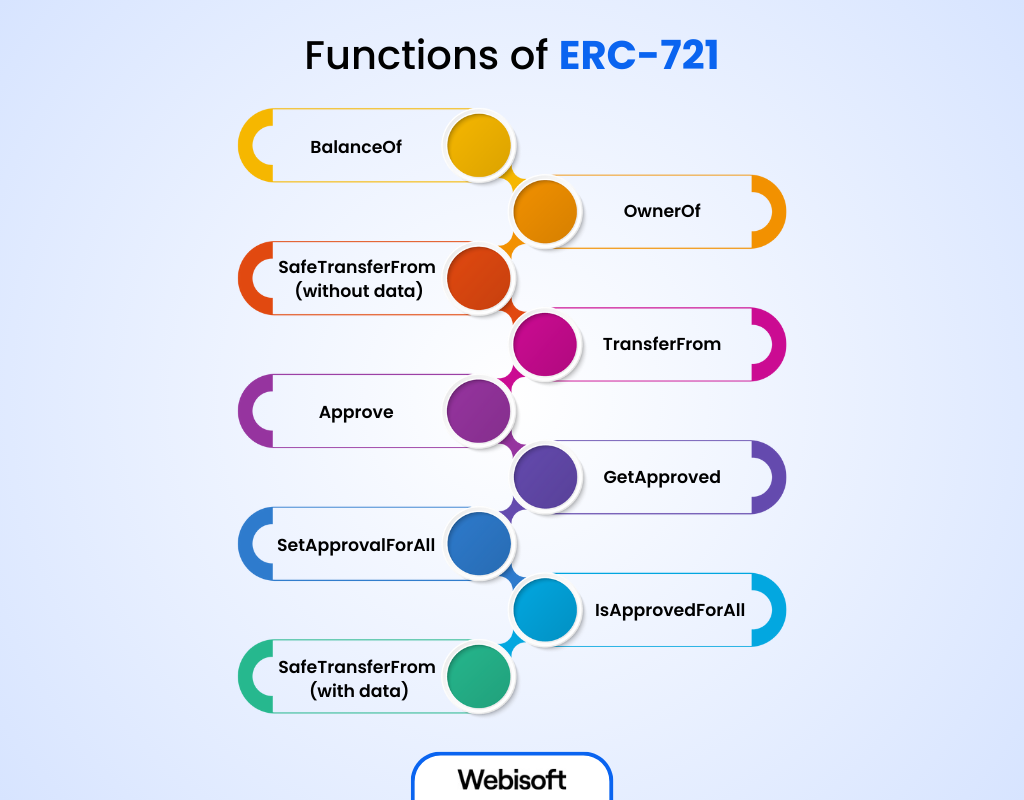
Let’s explore the key functions provided by ERC-721 for managing Non-Fungible Tokens (NFTs) on the Ethereum blockchain:
BalanceOf
This function provides the count of NFTs (Non-Fungible Tokens) owned by a specific address. It’s like counting how many unique digital items belong to a particular person.
OwnerOf
The ownerOf function helps identify the person who holds a specific token by checking its unique ID. It’s similar to finding out who owns a particular house by its unique address.
SafeTransferFrom (without data)
This function ensures the secure transfer of ownership for a specific token from one address to another. It also checks if the recipient is a smart contract and confirms whether it can accept the transfer.
TransferFrom
This function is used to move the ownership of a token from one address to another. It’s typically used when the sender has received permission to perform the transfer.
Approve
It approves a specific address for transferring a particular token. This feature enables trusted transfers, allowing an owner to authorize another party to handle the token on their behalf.
GetApproved
This function retrieves the address that has received approval for transferring a specific token. If there’s no approved address, it returns a null address, indicating that no permission has been granted.
SetApprovalForAll
This function empowers the owner of one or more tokens to approve or revoke approval for an operator to manage all of their tokens. It’s like appointing or dismissing a caretaker for an entire collection of digital assets.
IsApprovedForAll
This function checks whether an operator has approval to manage all tokens belonging to a particular owner. It verifies if the operator has the green light to oversee the entire collection.
SafeTransferFrom (with data)
Similar to the safeTransferFrom function without data, this function adds an extra data parameter. This parameter allows users to include additional information when transferring a token, especially when the recipient is a smart contract.
This function also performs checks to ensure the smart contract can handle the token. It implements the required onERC721Received function for a smooth transfer process.
How does the ERC-721 standard work?
The ERC-721 standard serves as a blueprint for developers. It incorporates specific functions into their smart contracts, enabling the creation, transfer, and management of Non-Fungible Tokens (NFTs).
These functions play an important role in the formation of unique tokens, each with its distinct metadata, ensuring their individuality.
ERC-721 smart contracts function as digital ledger keepers, diligently recording token ownership details. This ledger ensures the secure and transparent exchange of tokens among users. Moreover, it maintains a comprehensive account of the total token supply and the distribution of tokens among different addresses.
Benefits of Token ERC-721 for NFTs
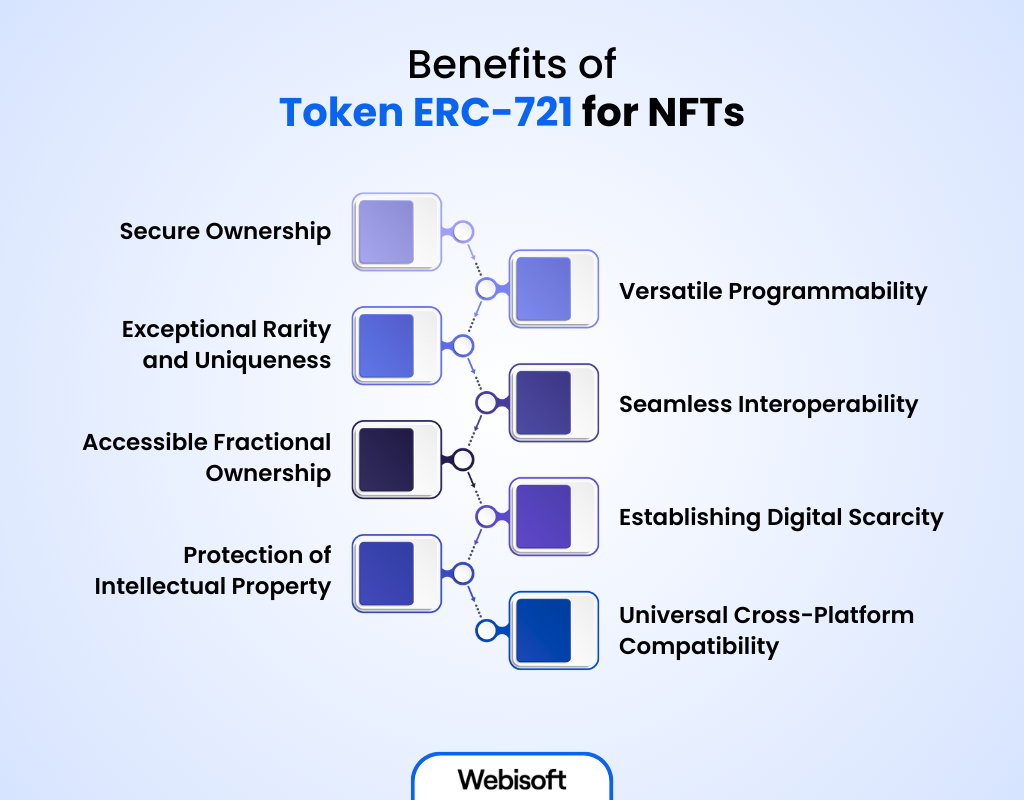
The ERC-721 token standard offers several significant benefits for Non-Fungible Tokens (NFTs). Here check out some of the benefits:
Secure Ownership
ERC-721 ensures that users can confidently own, transfer, and manage unique digital assets while maintaining transparent and verifiable records of ownership.
Versatile Programmability
ERC-721 provides creators with the ability to add extra features to NFTs. This includes functionalities like artist royalties, in-game utilities, or evolving attributes, broadening the range of potential applications.
Exceptional Rarity and Uniqueness
In contrast to interchangeable tokens, ERC-721 NFTs represent distinct items, each possessing its unique characteristics. This inherent uniqueness makes them highly valuable to collectors and creators.
Seamless Interoperability
NFTs following the ERC-721 standard can seamlessly work with various components of the Ethereum network, including marketplaces, wallets, and decentralized applications (dApps). This enhances their usability and accessibility.
Accessible Fractional Ownership
NFTs based on the ERC-721 standard can be divided into smaller, tradable parts, making it feasible for a wider audience to invest in high-value assets.
Establishing Digital Scarcity
With a limited supply and transparent history, ERC-721 NFTs create digital scarcity, which forms the basis for their intrinsic value and potential for long-term appreciation.
Protection of Intellectual Property
ERC-721 NFTs serve as guardians of intellectual property rights. They offer artists and creators an immutable ledger of their work and a tool to track its use and resale.
Universal Cross-Platform Compatibility
As they are built on the Ethereum network, ERC-721 NFTs function seamlessly across various platforms and applications. It opens up possibilities for innovative and diverse use cases.
What Are The Steps To Create and Deploy Erc 721 Tokens?
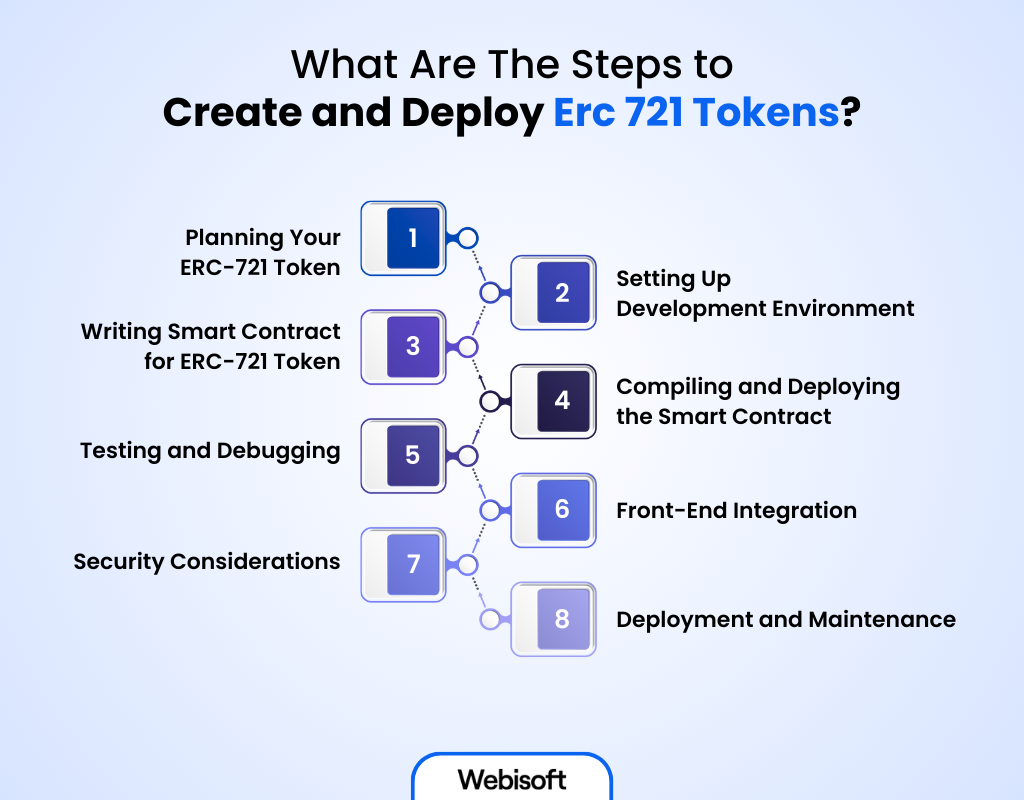
Here are the steps to create and deploy ERC-721 tokens, along with some example code snippets:
Step 1: Planning Your ERC-721 Token
Begin by thoroughly planning your ERC-721 token. Figure out why you want to create it and what it will be used for. Decide on its unique attributes like its name, symbol, and any extra information. Also, determine how many tokens will exist and how they’ll be owned and managed.
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/token/ERC721/ERC721.sol”;
contract MyERC721Token is ERC721 {
constructor() ERC721(“MyERC721Token”, “MET”) {
}
function mint(address to, uint256 tokenId, string memory tokenURI) external {
_mint(to, tokenId);
_setTokenURI(tokenId, tokenURI); }
}
Step 2: Setting Up Development Environment
To start creating your ERC-721 token, set up your workspace. This involves installing necessary tools like the Solidity compiler and Node.js. Create a project directory and configure it with the required settings.
Step 3: Writing Smart Contract for ERC-721 Token
Now, it’s time to write the actual code for your erc-721 smart contract. You’ll create a Solidity contract file with a .sol extension (e.g., “MyERC721Token.sol”). Import the necessary libraries, particularly the ERC-721 interface. Define your contract, inherit from the ERC-721 interface, and write the functions needed for token creation, transfer, and metadata management.
Here’s an example of a basic ERC-721 contract:
“`solidity
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/token/ERC721/ERC721.sol”;
contract MyERC721Token is ERC721 {
constructor() ERC721(“MyERC721Token”, “MET”) {
}
function mint(address to, uint256 tokenId) external {
_mint(to, tokenId);
}
}
“`
Step 4: Compiling and Deploying the Smart Contract
After writing the code, you need to compile it into a format that can run on the blockchain. Use the Solidity compiler for this. Then, deploy the contract onto a blockchain network, either a test network for initial testing or the Ethereum mainnet for real use. You can do this using tools like Truffle or Remix.
Step 5: Testing and Debugging
Thoroughly test your contract to make sure it works as intended. This includes creating tests to check if your contract can create and transfer tokens.
Unit tests:
pragma solidity ^0.8.0;
import “truffle/Assert.sol”;
import “truffle/DeployedAddresses.sol”;
import “../contracts/MyERC721Token.sol”;
contract TestMyERC721Token {
MyERC721Token token;
address public owner;
uint256 public tokenId;
function beforeAll() public {
token = new MyERC721Token();
owner = address(this);
tokenId = 1;
}
function testMintAndTransfer() public {
// Mint a token and transfer it to another address
address to = address(0x123);
token.mint(to, tokenId);
token.transferFrom(owner, to, tokenId);
// Check if the token owner is updated correctly
address tokenOwner = token.ownerOf(tokenId);
Assert.equal(tokenOwner, to, “Token owner should be the address ‘to’”);
}
}
Run these tests and fix any issues you encounter. Debugging tools can help you identify and resolve problems.
Step 6: Front-End Integration
To make your ERC-721 tokens accessible to users, create a user-friendly interface (UI). This UI should allow users to interact with your tokens. Connect this interface to your deployed smart contract using libraries like Web3.js or ethers.js.
import React, { useState } from “react”;
import { ethers } from “ethers”;
import MyERC721Token from “./contracts/MyERC721Token.json”;
function App() {
const [provider, setProvider] = useState(null);
const [contract, setContract] = useState(null);
async function connectWallet() {
if (window.ethereum) {
try {
await window.ethereum.request({ method: “eth_requestAccounts” });
const currentProvider = new ethers.providers.Web3Provider(window.ethereum);
const signer = currentProvider.getSigner();
setProvider(currentProvider);
const contractAddress = “YOUR_CONTRACT_ADDRESS”;
const contractAbi = MyERC721Token.abi;
const deployedContract = new ethers.Contract(contractAddress, contractAbi, signer);
setContract(deployedContract);
} catch (err) {
console.error(err);
}
} else {
console.error(“Please install MetaMask to connect your wallet.”);
}
}
async function mintToken() {
try {
const accounts = await provider.listAccounts();
const account = accounts[0];
const tokenId = 1;
const tokenURI = “https://example.com/token-metadata”;
await contract.mint(account, tokenId, tokenURI);
console.log(“Token minted successfully!”);
} catch (err) {
console.error(err);
}
}
return (
<div>
<button onClick={connectWallet}>Connect Wallet</button>
<button onClick={mintToken}>Mint Token</button>
</div>
);
}
export default App;
Implement features that let users create, transfer, and view token details.
Step 7: Security Considerations
Security is crucial. Identify potential security risks in your code and take steps to minimize them. Use best practices like controlling access, validating inputs, and designing your contract securely. You may want to get a professional security audit to ensure your contract is safe.
Step 8: Deployment and Maintenance
Before deploying your token ERC721 to the Ethereum mainnet, thoroughly test it. Once it’s ready, deploy it to the mainnet. After deployment, continue to monitor and maintain your contract to address any issues or updates that may come up over time. This ensures your ERC-721 token remains reliable and secure on the blockchain.
Please note that the provided code is a basic example, and you may need to add more functionality and error handling to suit your specific requirements. It’s also important to thoroughly test your contract and consider security best practices before deploying it to a production environment.
8 Use Cases for ERC-721 NFTs
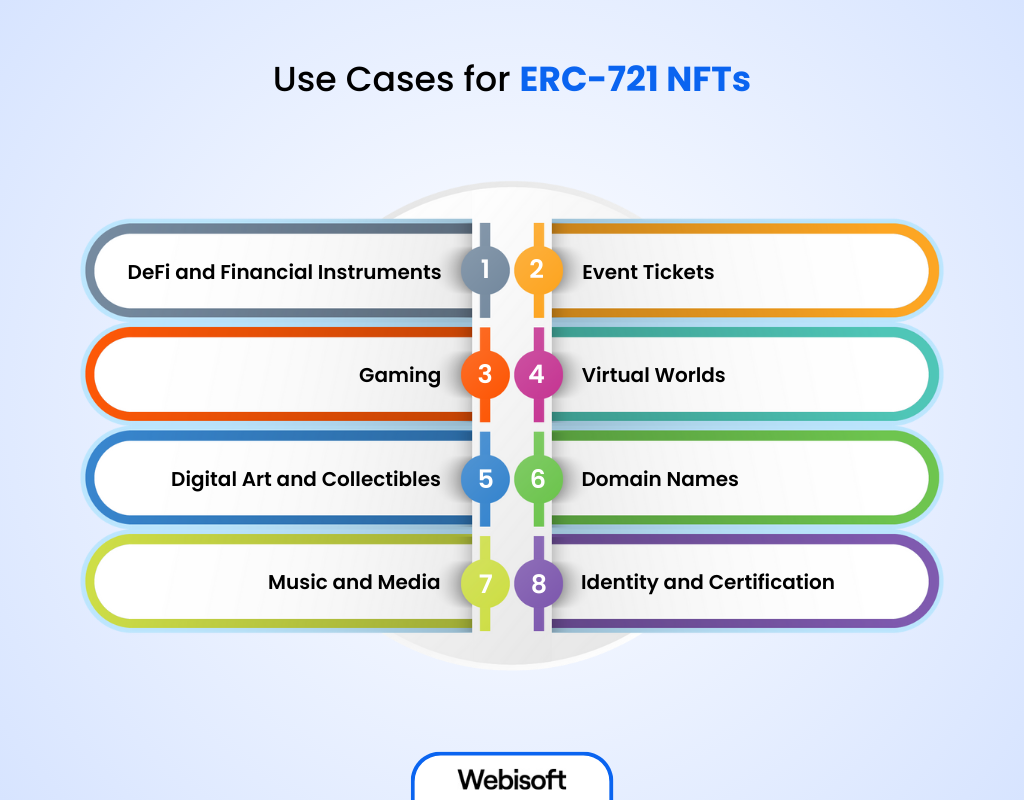
The ERC-721 token standard has opened up lots of possibilities across various industries. Let’s explore 8 innovative use cases of ERC-721 Non-Fungible Tokens (NFTs) in the following sections:
1. DeFi and Financial Instruments
In decentralized finance (DeFi), ERC-721 tokens enable the creation of unique financial products, like tokenized real estate, insurance policies, or bonds. This brings more flexibility and innovation to the financial sector, allowing for new and creative financial instruments.
2. Event Tickets
ERC-721 tokens can be used to create unique event tickets for concerts, conferences, and sports games. Each ticket is like a digital pass, and it can be securely transferred from one person to another, reducing the risk of fraud.
3. Gaming
ERC-721 tokens serve as the building blocks for blockchain-based games. In these games, each in-game item is unique and can be traded or sold among players. Think of them as the special collectibles or assets within the game.
4. Virtual Worlds
In virtual worlds like Decentraland and The Sandbox, ERC-721 tokens represent ownership of virtual land, buildings, and other assets. Users can buy, sell, and trade these assets in online marketplaces, similar to owning property in the real world.
5. Digital Art and Collectibles
NFTs have transformed the digital art world. They allow artists to sell their digital creations as unique tokens. Platforms like OpenSea, Rarible, and Art Blocks enable artists to create and sell their art as NFTs using the ERC-721 standard.
6. Domain Names
Some projects, like the Ethereum Name Service (ENS) and Unstoppable Domains, use ERC-721 tokens to represent domain names on the blockchain. This provides users with decentralized and censorship-resistant domain name ownership. Social platforms like Lens Protocol also use ERC-721 tokens for usernames.
7. Music and Media
Musicians and content creators can use ERC-721 tokens to turn their work into digital collectibles. This means fans can purchase and own special pieces of content, such as limited edition albums, videos, or exclusive merchandise. Some platforms, like EVEN, facilitate these transactions.
8. Identity and Certification
ERC-721 tokens can represent digital identities, educational certificates, or professional licenses. They offer a secure and tamper-proof way to store important credentials on the blockchain, ensuring their integrity.
Real-world examples of successful projects utilizing ERC-721 tokens
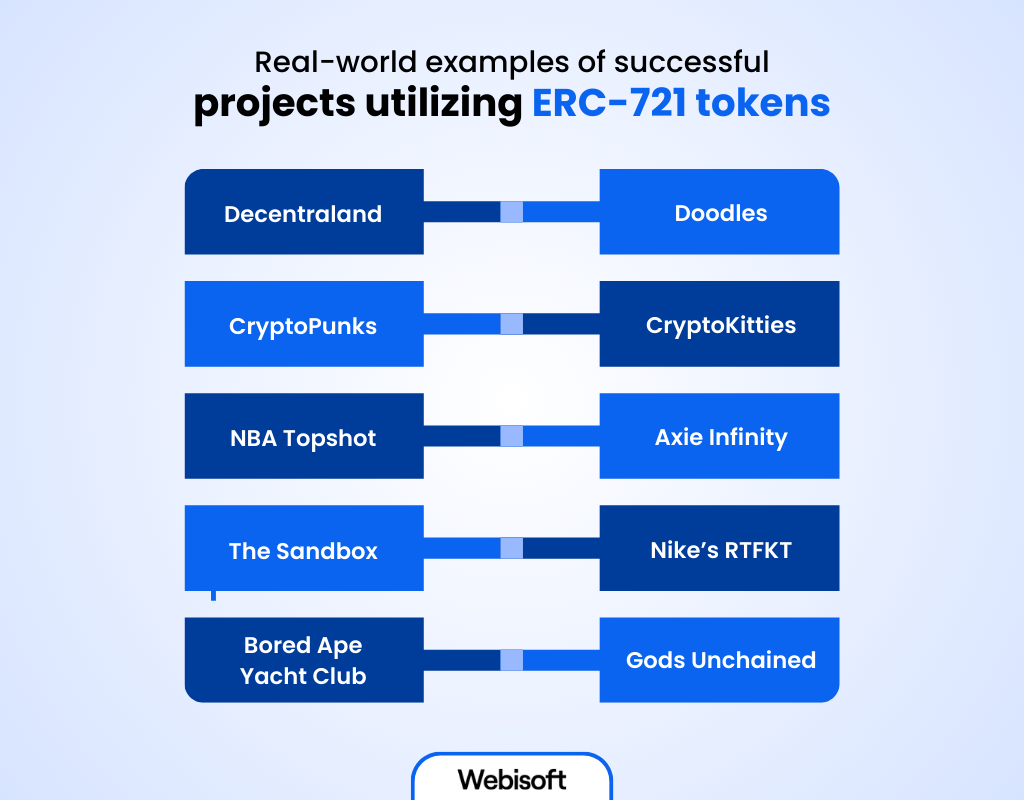
Let’s take a closer look at some real-world projects that have harnessed the power of ERC-721 tokens to create unique and innovative experiences:
Decentraland
This virtual world allows users to buy, develop, and trade virtual real estate and assets on the Ethereum blockchain. It’s like owning digital property in a vast online universe.
CryptoPunks
A collection of 10,000 unique pixel art characters, where each character is an ERC-721 token. These quirky characters have become sought-after digital collectibles.
NBA Topshot
Officially licensed by the NBA, Topshot lets basketball fans buy, sell, and trade officially licensed NBA collectible highlights. It’s a modern twist on sports memorabilia.
The Sandbox
In this virtual gaming world, users can create, own, and monetize their gaming experiences. It’s like a digital playground where creativity knows no bounds.
Bored Ape Yacht Club
An exclusive collection of 10,000 unique Bored Ape NFTs, each with its own set of traits and rarity. Owning one is like having a piece of digital art.
Doodles
Hand-drawn doodles transformed into unique NFT collectibles. It’s a fusion of traditional art and blockchain technology.
CryptoKitties
One of the pioneers of NFTs, CryptoKitties allows users to collect, breed, and trade unique virtual cats. Each cat is an ERC-721 token, making them one-of-a-kind digital pets.
Axie Infinity
This blockchain-based game lets players collect, breed, and battle fantasy creatures called Axies. It’s a unique blend of gaming and blockchain technology.
Nike’s RTFKT
The iconic sportswear brand Nike ventured into the NFT space, creating limited-edition digital sneakers. It’s a new way to own and showcase your favorite kicks.
Gods Unchained
A blockchain-based trading card game where players own and trade their cards as NFTs. It brings the concept of collectible card games into the digital age.
How to create NFT solidity With ERC-721?
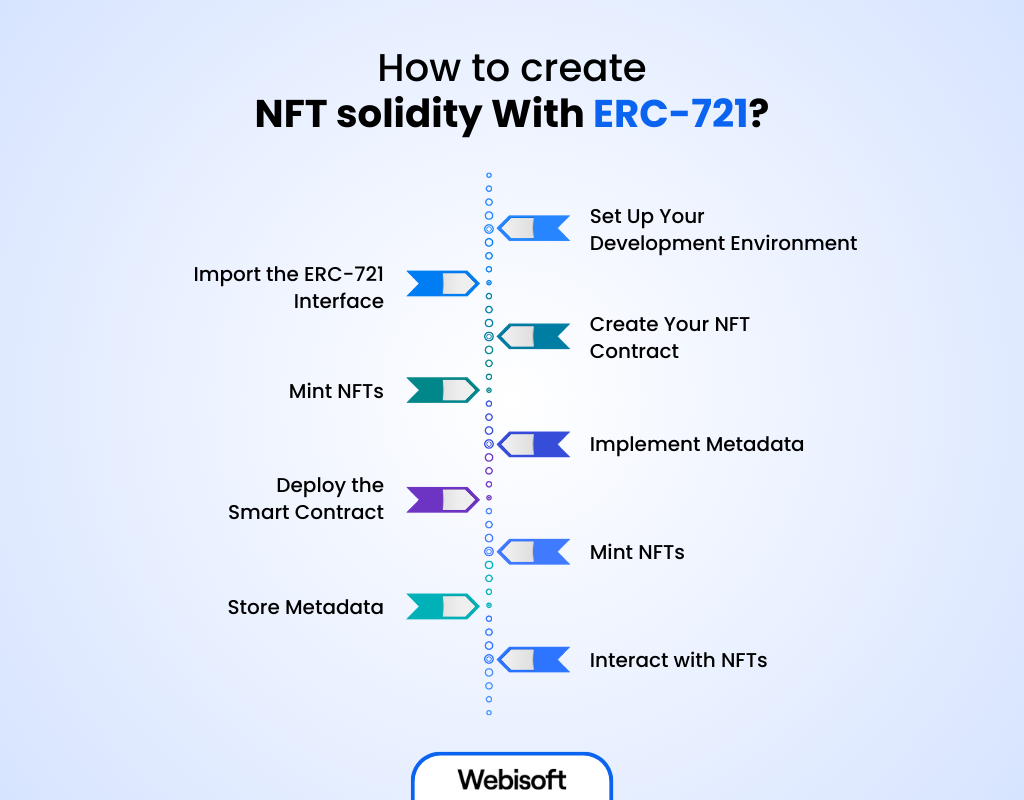
Creating an NFT (Non-Fungible Token) in Solidity using the ERC-721 standard involves writing a smart contract that complies with the ERC-721 interface. Below are the steps to create an NFT using Solidity:
Set Up Your Development Environment
Install a development environment like Remix, Truffle, or Hardhat.
Ensure you have a compatible Ethereum wallet for deploying and interacting with smart contracts.
Import the ERC-721 Interface
In your Solidity smart contract, start by importing the ERC-721 interface. You can do this with the following import statement:
import “@openzeppelin/contracts/token/ERC721/ERC721.sol”;
Make sure to install the OpenZeppelin library, which provides pre-built implementations of ERC standards.
Create Your NFT Contract
Define your NFT contract, inheriting from the ERC-721 standard. You’ll need to specify the name, symbol, and other parameters unique to your NFT.
contract MyNFT is ERC721 {
constructor() ERC721(“My NFT”, “MNFT”) {
}
}
Mint NFTs
To mint (create) NFTs, you’ll need a function in your contract. You can restrict minting to specific addresses (like the contract owner) or open it up to the public.
function mintNFT(address to, uint256 tokenId) public {
require(msg.sender == owner(), “Only the owner can mint NFTs”);
_mint(to, tokenId);
}
This function checks if the caller is the owner of the contract before minting the NFT.
Implement Metadata
You can store metadata associated with each NFT, such as its name, description, and external links. Use a mapping to associate token IDs with metadata.
mapping(uint256 => string) private _tokenURIs;
function setTokenURI(uint256 tokenId, string memory tokenURI) public {
require(_exists(tokenId), “Token does not exist”);
require(_isApprovedOrOwner(msg.sender, tokenId), “Not approved or owner”);
_tokenURIs[tokenId] = tokenURI;
}
function tokenURI(uint256 tokenId) public view returns (string memory) {
require(_exists(tokenId), “Token does not exist”);
string memory _tokenURI = _tokenURIs[tokenId];
return _tokenURI;
}
Deploy the Smart Contract
Deploy your smart contract to the Ethereum blockchain using Remix, Truffle, or a similar tool. Ensure you have enough ETH to cover gas fees for deployment.
Mint NFTs
Once deployed, you can mint NFTs by calling the mintNFT function, specifying the recipient’s address and a unique token ID.
Store Metadata
Store metadata off-chain, either on a centralized server or using decentralized storage solutions like IPFS. Associate the metadata URLs with token IDs using the setTokenURI function.
Interact with NFTs
Users can now interact with your NFTs by querying their metadata, transferring them to other addresses, or displaying them in NFT marketplaces and applications.
Remember to thoroughly test your smart contract on testnets before deploying it to the Ethereum mainnet. Additionally, consider security audits and best practices to ensure the safety of your NFTs and users’ assets.
How Webisoft Helps with erc721 token development and Implementation
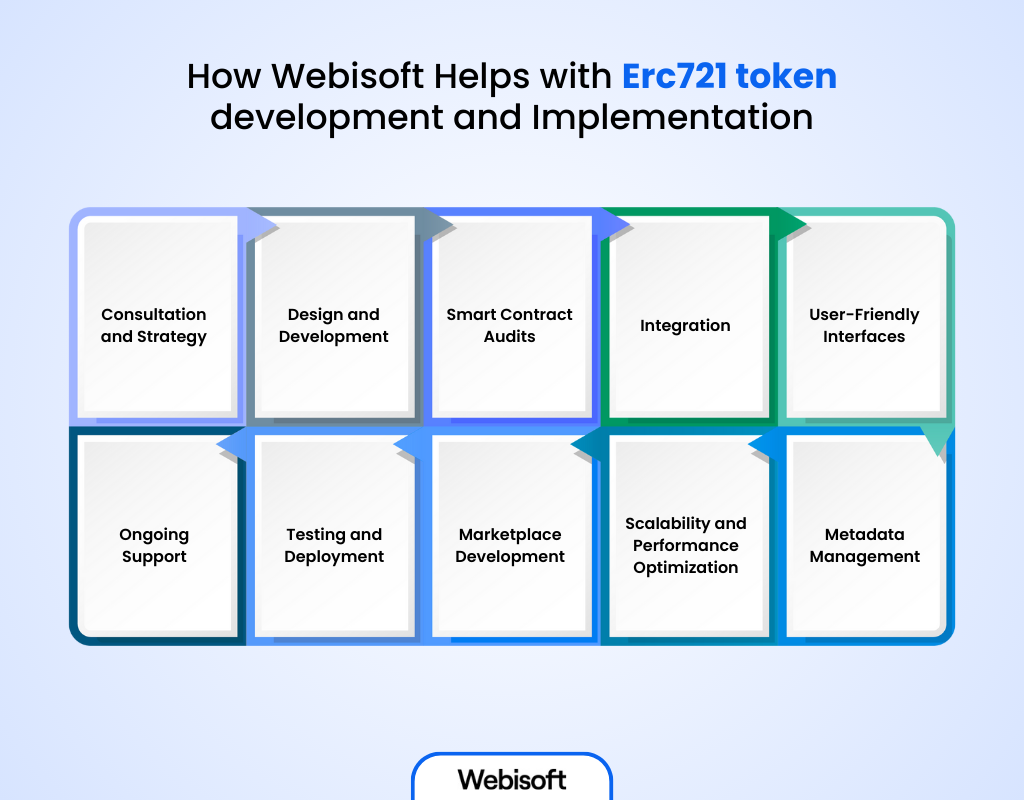
Webisoft provides comprehensive support and expertise for ERC-721 token development and implementation, making the process seamless and efficient. Here’s how Webisoft can assist you with ERC-721 token projects:
Consultation and Strategy
Webisoft begins by understanding your project requirements, goals, and business objectives. We provide expert consultation to determine the most suitable use cases and strategies for implementing ERC-721 tokens in your project.
Design and Development
Our experienced blockchain developers design and develop custom ERC-721 smart contracts tailored to your specific needs. We ensure compliance with ERC-721 standards and security best practices to protect your digital assets.
Smart Contract Audits
Security is paramount in blockchain development. Webisoft conducts thorough audits of your ERC-721 smart contracts to identify and rectify potential vulnerabilities, ensuring the safety of your NFTs and users’ assets.
Integration
We seamlessly integrate ERC-721 tokens into your existing applications or platforms, enabling you to leverage the benefits of non-fungible tokens in various industries, including art, gaming, real estate, and more.
User-Friendly Interfaces
Webisoft creates user-friendly interfaces and applications for your ERC-721 token ecosystem. This ensures a smooth user experience, making it easy for users to manage, trade, and interact with NFTs.
Metadata Management
We implement robust metadata management systems, allowing you to store and retrieve essential information related to your NFTs. This includes details about the token, its ownership, and associated media.
Scalability and Performance Optimization
Webisoft ensures that your ERC-721 token infrastructure is scalable to accommodate a growing user base. We optimize performance to handle high transaction volumes while maintaining responsiveness.
Marketplace Development
If your project involves creating an NFT marketplace or platform, we have the expertise to design, develop, and deploy the necessary components, including user interfaces, payment gateways, and search functionalities.
Testing and Deployment
Before deployment, we conduct extensive testing, including on testnets, to validate the functionality and security of your ERC-721 tokens. Once tested and audited, we assist with the deployment on the Ethereum mainnet or your desired blockchain network.
Ongoing Support
Webisoft also offers ongoing support, maintenance, and updates to ensure the long-term success of your ERC-721 token ecosystem.
Conclusion
To wrap up, in this article, we explored the features, benefits, and real-world applications of ERC 721 tokens, along with insights into their creation process. From secure ownership and versatile programmability to exceptional rarity and interoperability, ERC-721 tokens have showcased their potential across various industries.
As the NFT ecosystem continues to grow, ERC-721 tokens remain at the forefront of digital innovation, offering limitless possibilities for creators, collectors, and businesses.
If you are interested in the potential of ERC-721 tokens and eager to utilize them for your project. Reach out to Webisoft today. Explore how our expertise can guide you through NFT and reshape the future of digital ownership.
FAQs
Are ERC-721 tokens compatible with other blockchain networks besides Ethereum?
ERC-721 tokens are primarily designed for Ethereum and may require additional development for compatibility with other blockchains.
How can I ensure the uniqueness of my ERC-721 tokens in a large collection?
You can use a combination of token attributes, such as metadata or traits, to distinguish tokens within a collection.
Is it possible to change the metadata associated with an existing ERC-721 token?
The metadata of an ERC-721 token is typically immutable once set, but you can create a new token with updated metadata if needed.
Can ERC-721 tokens represent physical assets in the real world?
Yes, ERC-721 tokens can be used to represent ownership of physical assets through associated records and documentation.